SiteMap
is an XML file. You can use both the System.XML.Linq.XDocument and
System.XML.Linq.XElement classes to create XML. When you need to create
an XML document containing XML declaration, XML Document Type (DTD),
Processing instructions, Comments or Namespaces, you should go in for
the XDocument class. Here’s how to create and save a SiteMap file
dynamically using LINQ
Drag and drop a Button and a TreeView control from the toolbox to the Default.aspx page
<body>
<form id="form1" runat="server">
<div>
<asp:Button ID="btnCreate" runat="server"
Text="Create and Bind" />
<br />
<br />
<asp:TreeView ID="TreeView1" runat="server">
</asp:TreeView>
</div>
</form>
</body>
Now add the following code on the click event of the button
C#
protected void btnCreate_Click(object sender, EventArgs e)
{
XNamespace siteNM = "http://schemas.microsoft.com/AspNet/SiteMap-File-1.0";
XDocument xDoc = new XDocument(
new XDeclaration("1.0", "UTF-8", null),
new XElement(siteNM + "siteMap",
new XElement(siteNM + "siteMapNode", new XAttribute("title", "My Favorites"),
new XElement(siteNM + "siteMapNode", new XAttribute("title", "Favorite Sites"),
new XElement(siteNM + "siteMapNode", new XAttribute("title", "ASP.NET Home"), new XAttribute("url", "http://www.asp.net")),
new XElement(siteNM + "siteMapNode", new XAttribute("title", "ASP.NET Articles"), new XAttribute("url", "http://www.dotnetcurry.com")),
new XElement(siteNM + "siteMapNode", new XAttribute("title", "Windows Client"), new XAttribute("url", "http://www.windowsclient.net")),
new XElement(siteNM + "siteMapNode", new XAttribute("title", "Silverlight"), new XAttribute("url", "http://silverlight.net"))
),
new XElement(siteNM + "siteMapNode", new XAttribute("title", "Favorite Blogs"),
new XElement(siteNM + "siteMapNode", new XAttribute("title", "ScottGu Blog"), new XAttribute("url", "http://weblogs.asp.net/scottgu"),
new XElement(siteNM + "siteMapNode", new XAttribute("title", "Technology Blog"), new XAttribute("url", "http://www.devcurry.com")),
new XElement(siteNM + "siteMapNode", new XAttribute("title", "SQL Blog"), new XAttribute("url", "http://www.sqlservercurry.com")),
new XElement(siteNM + "siteMapNode", new XAttribute("title", "Food Lovers"), new XAttribute("url", "http://foodatarian.com"))
)) )
));
// Save to Disk
xDoc.Save(Server.MapPath("web.sitemap"));
}
VB.NET
Protected Sub btnCreate_Click(ByVal sender As Object, ByVal e As System.EventArgs)
Dim siteNM As XNamespace = "http://schemas.microsoft.com/AspNet/SiteMap-File-1.0"
Dim xDoc As New XDocument(New XDeclaration("1.0", "UTF-8", Nothing), _
New XElement(siteNM + "siteMap", _
New XElement(siteNM + "siteMapNode", New XAttribute("title", "My Favorites"), _
New XElement(siteNM + "siteMapNode", New XAttribute("title", "Favorite Sites"), _
New XElement(siteNM + "siteMapNode", New XAttribute("title", "ASP.NET Home"), New XAttribute("url", "http://www.asp.net")), _
New XElement(siteNM + "siteMapNode", New XAttribute("title", "ASP.NET Articles"), New XAttribute("url", "http://www.dotnetcurry.com")), _
New XElement(siteNM + "siteMapNode", New XAttribute("title", "Windows Client"), New XAttribute("url", "http://www.windowsclient.net")), _
New XElement(siteNM + "siteMapNode", New XAttribute("title", "Silverlight"), New XAttribute("url", "http://silverlight.net"))), _
New XElement(siteNM + "siteMapNode", New XAttribute("title", "Favorite Blogs"), _
New XElement(siteNM + "siteMapNode", New XAttribute("title", "ScottGu Blog"), New XAttribute("url", "http://weblogs.asp.net/scottgu"), _
New XElement(siteNM + "siteMapNode", New XAttribute("title", "Technology Blog"), New XAttribute("url", "http://www.devcurry.com")), _
New XElement(siteNM + "siteMapNode", New XAttribute("title", "SQL Blog"), New XAttribute("url", "http://www.sqlservercurry.com")), _
New XElement(siteNM + "siteMapNode", New XAttribute("title", "Food Lovers"), New XAttribute("url", "http://foodatarian.com")))))))
' Save to Disk
xDoc.Save(Server.MapPath("web.sitemap"))
End Sub
Note:
As you might have observed, I have added a namespace for each element.
Here all elements use one namespace. In case you don't add a namespace
for one parent element, it will add a empty namespace (xmlns=””) and the
compiler will raise a warning “The element 'siteMap' in namespace
'http://schemas.microsoft.com/AspNet/SiteMap-File-1.0' has invalid child
element 'siteMapNode’ “. Hence the trick is to add a namespace for each
element.
Once
the SiteMap is created, in order to bind it to the TreeView, all that
is needed is to create a SiteMapDataSource and bind it to the TreeView
C#
...
// Save to Disk
xDoc.Save(Server.MapPath("web.sitemap"));
if(File.Exists(Server.MapPath("web.sitemap")))
{
TreeView1.DataSource = new SiteMapDataSource();
TreeView1.DataBind();
}
VB.NET
...
' Save to Disk
xDoc.Save(Server.MapPath("web.sitemap"))
If File.Exists(Server.MapPath("web.sitemap")) Then
TreeView1.DataSource = New SiteMapDataSource()
TreeView1.DataBind()
End If
The result is a dynamically created SiteMap bound to a TreeView control
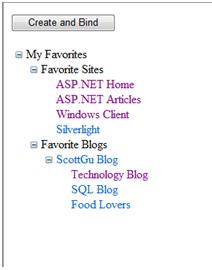
No comments:
Post a Comment