Tip 1: Change the style of the selected page or highlight the current page in the GridView Pager control
A simple way is to use CSS as shown below. The <PagerStyle> is set to a css class which modifies the style of the pager:
<head runat="server">
<title></title>
<style type="text/css">
.cssPager span { background-color:#4f6b72; font-size:18px;}
</style>
</head>
<asp:GridView ID="GridView1" runat="server" AllowPaging="true" AutoGenerateColumns="false" DataKeyNames="ProductID" DataSourceID="SqlDataSource1">
<PagerStyle CssClass="cssPager" />
...
The output is as shown below, with the style set for the current page.
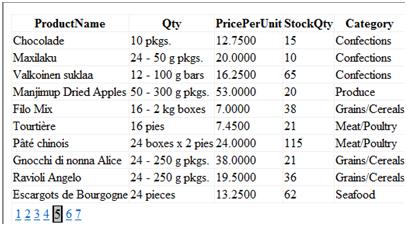
Tip 2: How to increase the spacing between the ASP.NET GridView Pager Numbers
One
simple way is to use CSS again. Observe how we have set a cssClass to
the PagerStyle, similar to what we saw in Tip 1, and are increasing the
padding for the <td>:
<head runat="server">
<title></title>
<style type="text/css">
.cssPager td
{
padding-left: 4px;
padding-right: 4px;
}
</style>
</head>
<asp:GridView ID="GridView1" runat="server" AllowPaging="true" AutoGenerateColumns="false" DataKeyNames="ProductID" DataSourceID="SqlDataSource1">
<PagerStyle CssClass="cssPager" />
...
The output looks similar to the following with the increased space between the page number display:
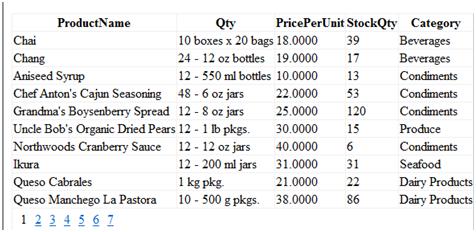
Tip 3: Display GridView Paging even for a single page
If we change the query of the SQLDataSource to “SELECT
TOP 10 [ProductID], [ProductName], [QuantityPerUnit], [UnitPrice],
[UnitsInStock], [CategoryName] FROM [Alphabetical list of products]”
we get only 10 rows of data. If you view the application, you will
observe that there isn’t a pager displayed, since the number of pages
displayed is just one(remember that the pagesize is set to 10). In order
to display the pager row even for one page, use this code in the
PreRender event of the GridView which sets the visibility of the
BottomPagerRow to true.
C#
protected void GridView1_PreRender(object sender, EventArgs e)
{
GridView gv = (GridView)sender;
GridViewRow gvr = (GridViewRow)gv.BottomPagerRow;
if (gvr != null)
{
gvr.Visible = true;
}
}
VB.NET
Protected Sub GridView1_PreRender(ByVal sender As Object, ByVal e As EventArgs)
Dim gv As GridView = CType(sender, GridView)
Dim gvr As GridViewRow = CType(gv.BottomPagerRow, GridViewRow)
If gvr IsNot Nothing Then
gvr.Visible = True
End If
End Sub
Tip 4: Add a Label Control at the GridView footer to display page count
The first step is to set the ‘ShowFooter’ property of the GridView to ‘true’
Then in the GridView1_RowDataBound, add the following code:
C#
protected void GridView1_RowDataBound(object sender, GridViewRowEventArgs e)
{
if (e.Row.RowType == DataControlRowType.Footer)
{
e.Row.Cells[0].Text = "Page " + (GridView1.PageIndex + 1) + " of " + GridView1.PageCount;
}
}
VB.NET
Protected Sub GridView1_RowDataBound(ByVal sender As Object, ByVal e As GridViewRowEventArgs)
If e.Row.RowType = DataControlRowType.Footer Then
e.Row.Cells(0).Text = "Page " & (GridView1.PageIndex + 1) & " of " & GridView1.PageCount
End If
End Sub
The result is displayed below – the blue colored block with the page count, showing Page 4 of 7.
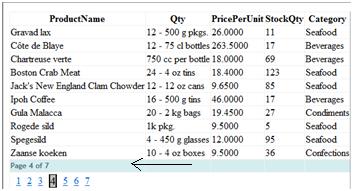
Tip 5: Create a custom pager template with paging using a DropDownList control
Instead of using the built in Paging UI, if you want to define your own UI, use the <PagerTemplate>.
In
this sample, we will keep a dropdownlist control to select pages
instead of the default pager row UI. We will navigate pages of the
GridView using the dropdownlist.
Add a <PagerTemplate> to the GridView as shown below:
<asp:GridView ID="GridView1" runat="server" AllowPaging="true" AutoGenerateColumns="false"
DataKeyNames="ProductID" DataSourceID="SqlDataSource1"
ShowFooter="False" OnDataBound="GridView1_DataBound">
<PagerTemplate>
<table width="100%">
<tr>
<td style="width:70%">
<asp:DropDownList ID="ddlPaging" runat="server" AutoPostBack="true"
OnSelectedIndexChanged="ddlPaging_SelectedIndexChanged" />
</td>
</tr>
</table>
</PagerTemplate>
If
you observe, we have added the OnDataBound event to the GridView. This
is where we populate the DropDownList control with page data as shown
below:
C#
protected void GridView1_DataBound(object sender, EventArgs e)
{
DropDownList ddl = (DropDownList)GridView1.BottomPagerRow.Cells[0].FindControl("ddlPaging");
for (int cnt = 0; cnt < GridView1.PageCount; cnt++)
{
int curr = cnt + 1;
ListItem item = new ListItem(curr.ToString());
if (cnt == GridView1.PageIndex)
{
item.Selected = true;
}
ddl.Items.Add(item);
}
}
VB.NET
Protected Sub GridView1_DataBound(ByVal sender As Object, ByVal e As EventArgs)
Dim ddl As DropDownList = CType(GridView1.BottomPagerRow.Cells(0).FindControl("ddlPaging"), DropDownList)
For cnt As Integer = 0 To GridView1.PageCount - 1
Dim curr As Integer = cnt + 1
Dim item As New ListItem(curr.ToString())
If cnt = GridView1.PageIndex Then
item.Selected = True
End If
ddl.Items.Add(item)
Next cnt
End Sub
Since
the paging is now to be done using the DropDownList, we need a
mechanism where the pageindex of the GridView is set, whenever the user
selects a item in the DropDownList control. We need to handle the
OnSelectedIndexChanged for this purpose.
C#
protected void ddlPaging_SelectedIndexChanged(object sender, EventArgs e)
{
DropDownList ddl = (DropDownList)GridView1.BottomPagerRow.Cells[0].FindControl("ddlPaging");
GridView1.PageIndex = ddl.SelectedIndex;
}
VB.NET
Protected Sub ddlPaging_SelectedIndexChanged(ByVal sender As Object, ByVal e As EventArgs)
Dim ddl As DropDownList = CType(GridView1.BottomPagerRow.Cells(0).FindControl("ddlPaging"), DropDownList)
GridView1.PageIndex = ddl.SelectedIndex
End Sub
Shown below is the output:
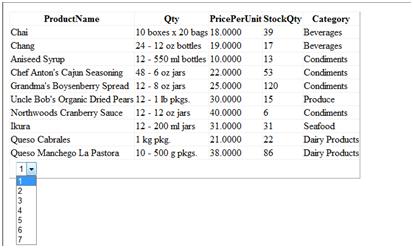
No comments:
Post a Comment